Python lists are used to store multiple items or objects under a single variable.
Lists are the built-in data types in Python whose function is to store data.
As we are already familiar with the concept of tuples, sets and dictionaries in Python whose function is also like that of the lists.
They are declared within square braces.
Lists are ordered, changeable, and allows duplication.
numbers = ["1","2", "3", "4"] print((numbers))
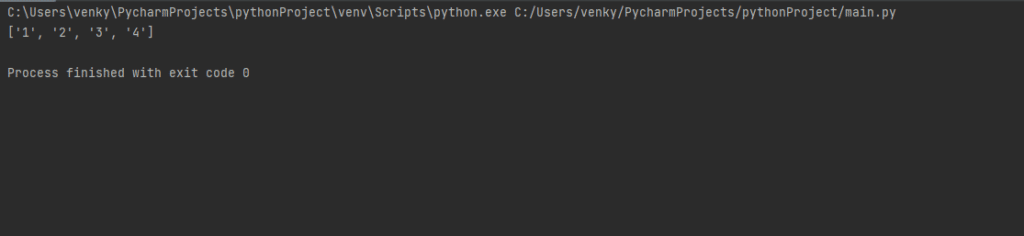
Determining the length of the list:
We can determine the length of list by using len() in the coding.
numbers = ["1", "2", "3", "4"] print(len(numbers))
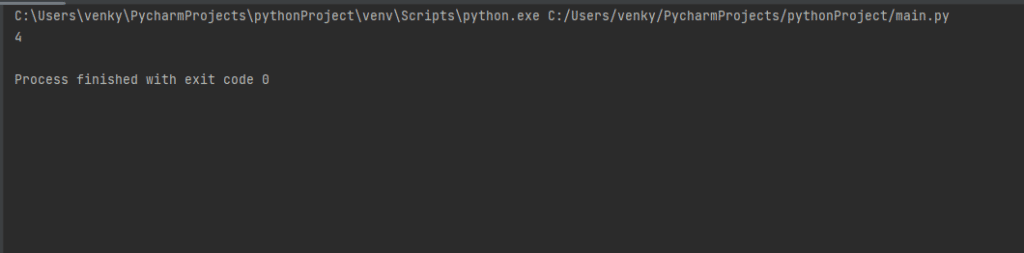
Data Types:
List items can take in any data type like string, int, etc.
listprequel = ["2","True","String","1.234"] print(listprequel)

Multiple lines of coding:
What if we want only a specific block of code after coding multiple lines?
It is simple and possible in python what we got to do is manipulations let us see the coding now:
listprequel1 = ["2", "True", "1.234", "string"] listprequel2 = ["2", "3", "4"] list3 = ["$", "%", "^"] print(list3)
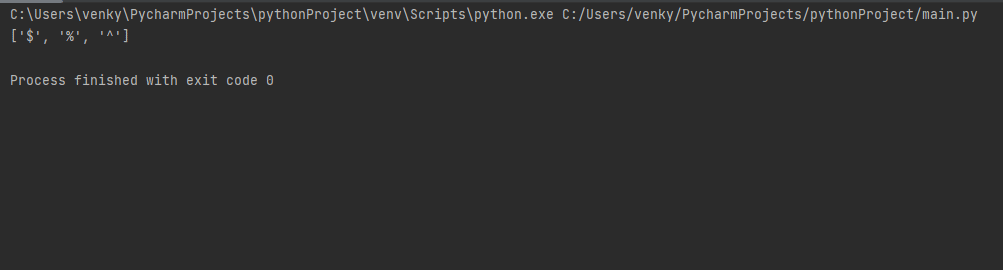
Indexing:
In coding we have the indexed value from [0] next [1]
numbers = ["2","3","4","5"] print(numbers[0])
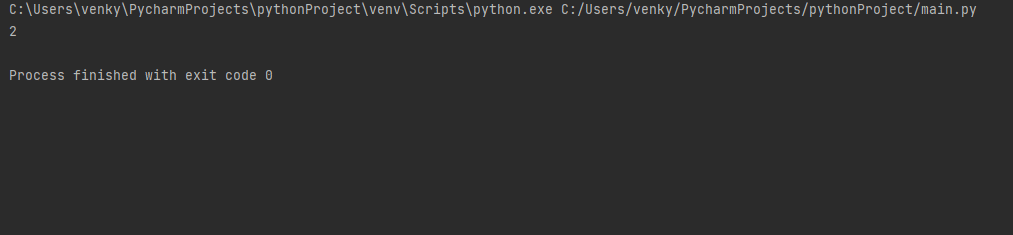
Range of the index:
What is range of an index?
Say we have five items enclosed within our list and we want only 2 items then here we use the range of index which will give us only the ones which the user desires to get.
Now let us see the coding:
cars = ["volvo", "bmw", "ford", "honda", "tata"] print(cars[2:5])
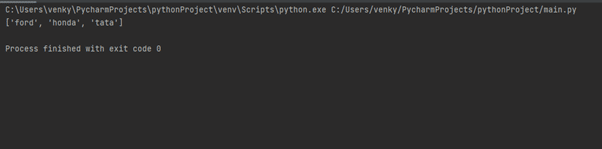
now if we want to get all the cars in the output then:
cars = ["volvo", "bmw", "ford", "honda", "tata"] print(cars[:5])
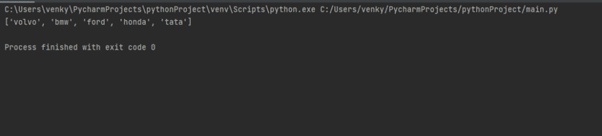
now if we want to exclude volvo bmw and print the rest:
cars = ["volvo", "bmw", "ford", "honda", "tata"] print(cars[2:5])
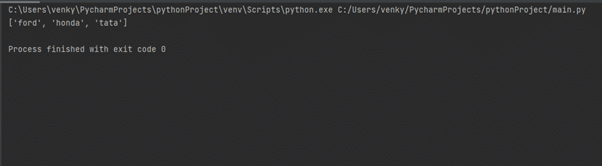
Negative indexing:
If we want to change the order of a specific list and then want only a single output then we got to count the numbers from right to left.
Next what?
Let us see the code to understand the previous lines.
numbers = ["2","3","4","5"] print(numbers[-1])
How do we access in the negative format?
If we want to print only some of the negative indexed items then we need to code accordingly.
cars = ["volvo", "bmw", "ford", "honda", "tata"] print(cars[-3:-1])
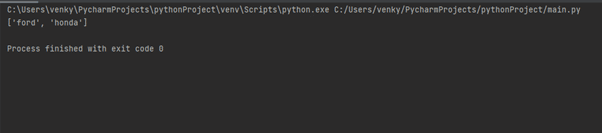
Check whether an item exist in the list or not:
cars = ["volvo", "bmw", "ford", "honda", "tata"] if "bmw" in cars: print("i'm available")
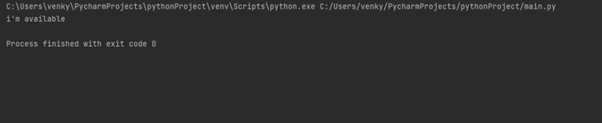
Adding a specific item to the list?
We can add the item in between the list as we are already familiar with the definition that lists are changeable so you can do some manipulations to the code and get it trot.
cars = ["volvo", "bmw", "ford", "honda", "tata"] cars.append("hyundai") print(cars)
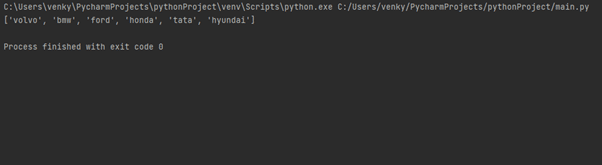
How to change the order of an item by other?
It is simple what we got to do is just use the insert keyword.
cars = ["volvo", "bmw", "ford", "honda", "tata"] cars.insert(1, "hyundai") print(cars)
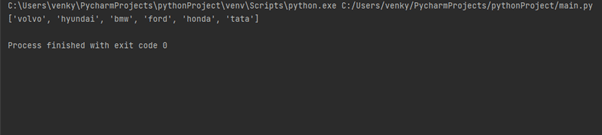
How to invoke the items to the list existing?
We need to just use the extend and get the desired output:
cars = ["volvo", "bmw", "ford", "honda", "tata"] year = ["1954", "1989", "1970"] cars.extend(year) print(cars)
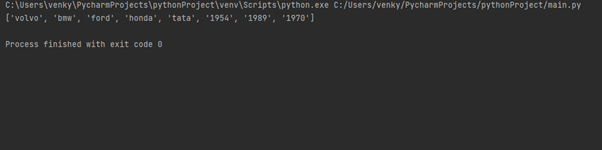
How to remove something from a list??
When we want to remove any of the item in the list?
There are there four methods by which we can remove something from our list they are:
- using remove keyword
- using pop() keyword
- using del keyword
- using clear keyword
It can be done simply using the keyword remove in the coding:
cars = ["volvo", "bmw", "ford", "honda", "tata"] cars.remove("bmw") //removes the specified object by variable name print(cars)
If we wanted to remove a specific index item from the list then pop() can be used.
cars = ["volvo", "bmw", "ford", "honda", "tata"] cars.pop(0) //removes the specific object by indexing.. print(cars)
What is the difference between pop() and remove ?
Using remove keyword what we are going to do is simply specify the element which we want to remove and eliminate it in the case of pop() what we do is eliminate the item from the list and return to it by declaring it at the time of declaration..
Confusing right let us see the code for the same..
cars = ["volvo", "bmw", "ford", "honda", "tata"] x = cars.pop(0) //removes the specific object by indexing.. print(cars) print(x) //by declaring this we can return to the element which we have removed..
del keyword removes a specific index let us see how?
cars = ["volvo", "bmw", "ford", "honda", "tata"] del cars[0] print(cars)
using del keyword the user will be benefited where in we can remove a range of elements from the list at once let us see the coding for the same which gives you a clarity …
cars = ["volvo", "bmw", "ford", "honda", "tata"] del cars[0:3] print(cars)
clear is used to wipe off the whole index.
cars = ["volvo", "bmw", "ford", "honda", "tata"] cars.clear() print(cars)