Generally a Boolean expression gives user a clarity whether a assigned variable is true or false.
Now let us discuss it in more detail by considering an example.
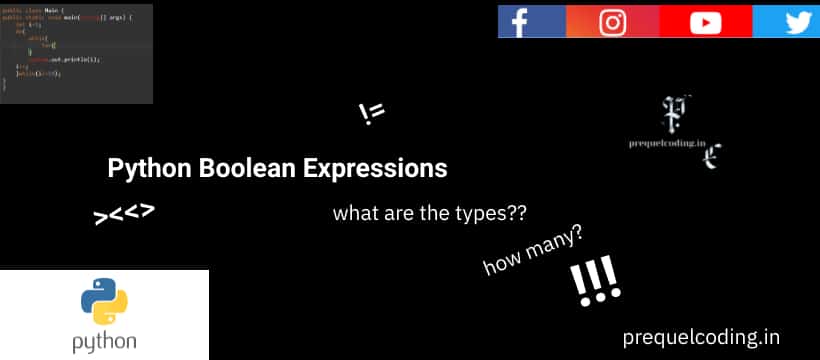
Say there are two friends and let us compare their heights.
now say person 1 is taller than person 2.
Let us try to now put this in a code language.
person 1== person 2
In this case the response might be true if person 1 is taller than the second person.
then we say person 1 > person 2
Or may be false if we assume that person 1 is shorter than the person 2.
This is how Boolean expression implementation takes place if in case the response is true then we continue using looping statements in case if false then we end looping..
Let us look at some of the basic Boolean Expressions:
Expression | Function |
> | greater than |
< | less than |
>= | greater than or equal to |
<= | less than or equal to |
!=/== | not equal to/equal to |
<> | alternate expression for not equal to |
Let us try to implement the code and see how boolean statements work.
less than expression:
a = 3; if a < 3: print("true") else: print("false")
output:
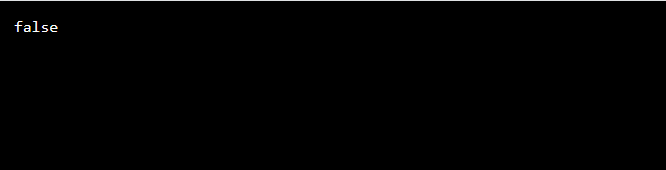
greater than expression:
a = 3; if a > 3: print("true") else: print("false")
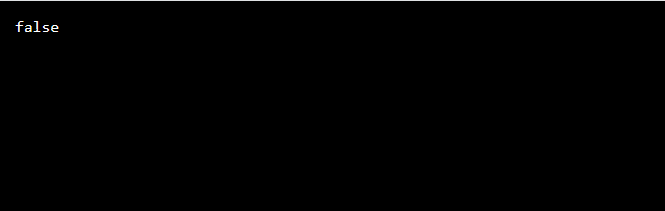
greater than or equal to:
a = 3; if a >= 3: print("true") else: print("false")
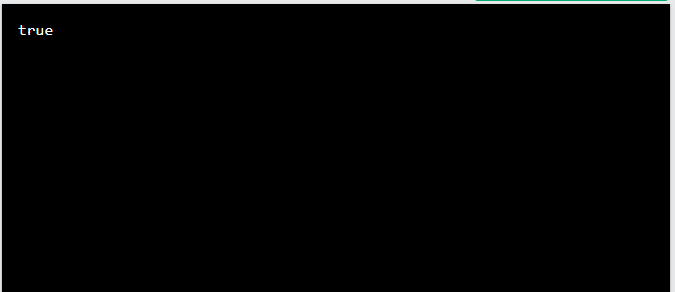
less than or equal to:
a = 3; if a <= 3: print("true") else: print("false")
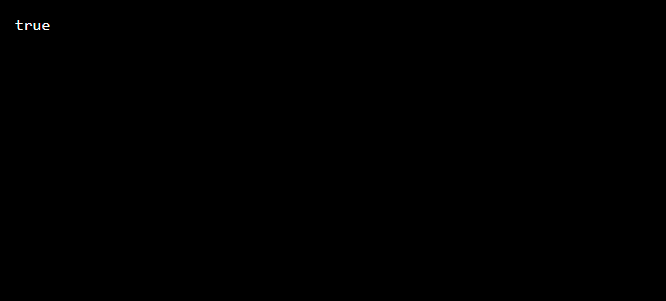
equal to:
a = 20 if a == 20: print("true") else: print("false")
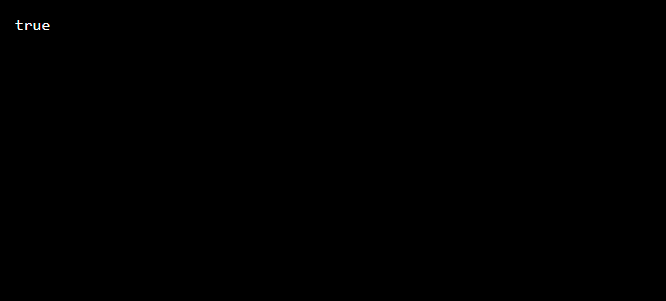
not equal to:
a = 20 if a != 2: print("true") else: print("false")
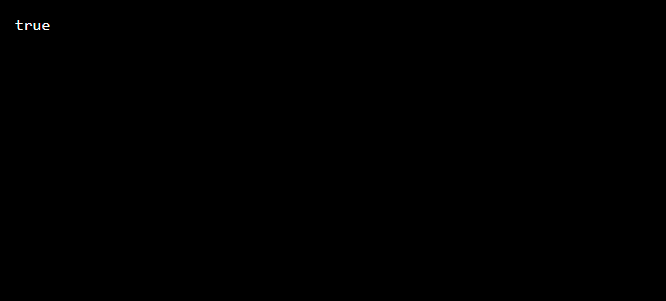