In this blog we will look at the palindrome program in java this consists of two parts:
- Palindrome check for a String
- Palindrome check for a Number
Now let us get started with how do we check for a palindrome string in java:
import java.util.Scanner;
public class Main{
public static boolean isPalindrome(String str){
int start = 0;
int end = str.length() - 1;
while(start<end){
if(str.charAt(start)!= str.charAt(end)){
return false;
}
start++;
end--;
}
return true;
}
public static void main(String[] args){
Scanner s = new Scanner(System.in);
String str = s.next();
if(isPalindrome(str)){
System.out.println("Palindrome");
}
else{
System.out.println("Non palindrome");
}
}
}
Now let us look at the number palindrome check:
public class Main
{
public static void Main(String[] args
{
variable initialisation:
int r, sum = 0, temp;
int n = 121;
temp = n;
invoking loops:
while(n>0){
r = n%10;
sum = (sum*10 + r);
n = n/10;
}
testing condition:
if(temp == sum){
System.out.println("palindrome");
}
else
System.out.println("non palindrome");
}
}
//Full code:
public class Main
{
public static void main(String[] args) {
int r, sum = 0, temp;
int n = 121;
temp = n;
while(n>0){
r = n%10;
sum = (sum*10 + r);
n = n/10;
}
if(temp == sum){
System.out.println("palindrome");
}
else
System.out.println("non palindrome");
}
}
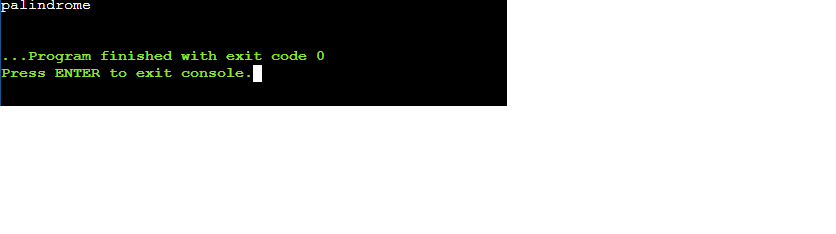