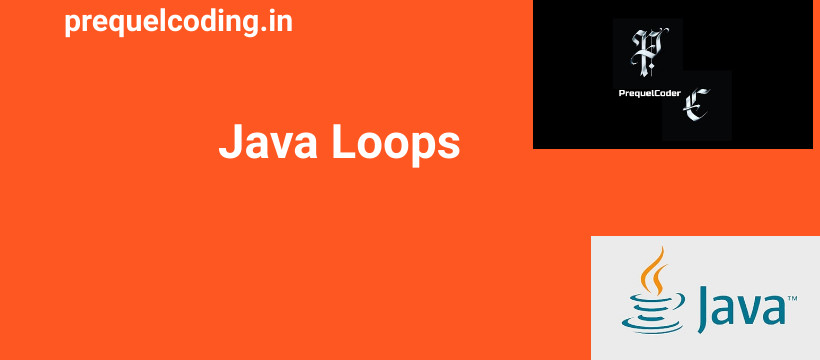
In this blog we will dive into the concept of looping in Java.
What is a Loop?
Loop in general is a set of sequence of instruction which is repeated until a condition is reached.
Why do we use loops in Java?
Loops in Java come into play when have a block of statements or code to be repeated.
We have loops classified into three types in Java:
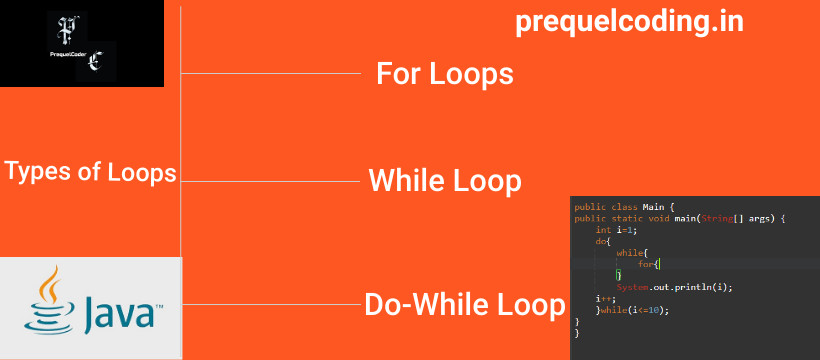
- For Loop
- While Loop
- Do-while Loop
Let us discuss about each of these loops in detail.
For Loop:
For Loop in Java is an entry-controlled loop which is used to execute a block of statements iteratively for a fixed number of times.
Now let us try to understand for loop concept more clearly with an example:
Code:
public class Main {
public static void Main (String [] args) {
for (int i = 0; i <= 10; i = i +2) {
System.out.println(i);
}
} }
// output :
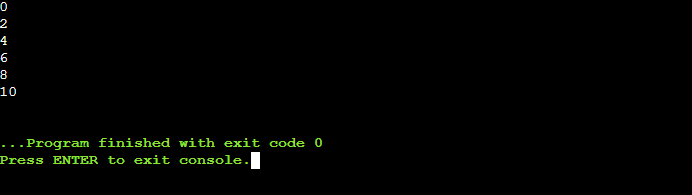
The above image is the output for the for loop code.
While Loop:
While loop is used to iterate a block of code until the Boolean condition turns out to be true. As soon as the Boolean condition becomes false the loops ends automatically.
Now let us try to understand this with an example:
Code:
public class Main {
public static void main(String [] args) {
int i = 0;
while( i<=10) {
System.out.println(i);
i++;
}
}
}
//output
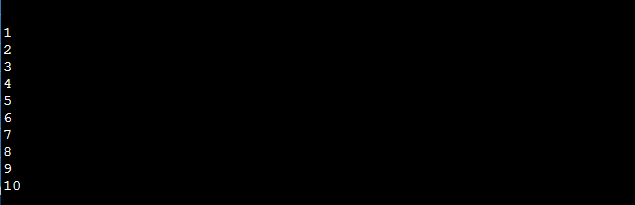
Do-While Loop:
The do-while loops in java comes into play when we want to iterate a block of code until the condition turns out to be true. If the number of iterations are not fixed then we have to execute atleast once where the do-while loops can be used.
The do-while loop is also called as the exit loop. Therefore, unlike the for loop and while loop we execute this loop at end of the program.
Now let us understand more clearly with an example:
Code: public class Main { public static void main(String[] args) { int i=1; do{ System.out.println(i); i++; }while(i<=10); } } //output:
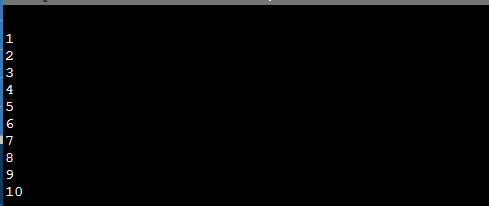
This is the concepts of looping in Java.
And this comes to the end of the concept of basic looping in Java and also end of this blog.
For more interesting content do follow and subscribe to us on our social platforms.