Type Casting is used for converting a primitive data type to another type.
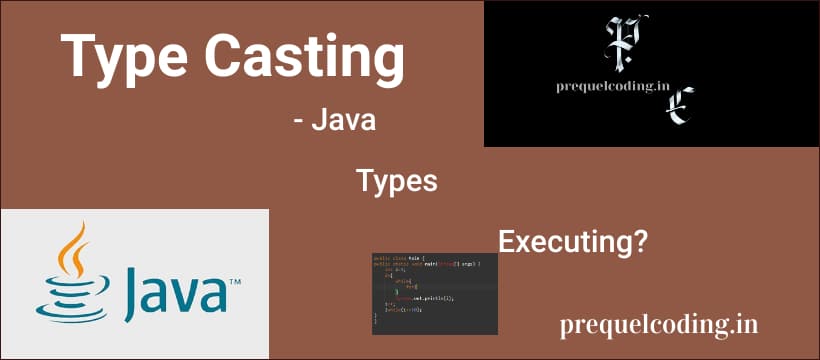
For example, we have a requirement to convert an int data type to a double data type we can perform it with the help of type casting.
Java provides us with two types of casting:
1. Widening Casting
2. Narrow Casting
Widening Casting:
It is a type of casting where we can convert a small data type to a larger data type automatically.
Let us see how:
If we have a requirement to convert an int to double:
We just need to declare an int variable first followed by the double let us see how.
int myInt = 9;
double myDouble = (myInt); Full code: public class Main { public static void main(String[] args) { int myInt = 9; double myDouble = (myInt); System.out.println(myInt); System.out.println(myDouble); } }
Narrow Casting:
It is a type casting which we use to convert a small data type to a larger data type manually by adding parenthesis.
Let us see how:
Now let me just declare a double followed by int datatype.
double myDouble = 9.5d;
int myInt = (int)myDouble; Full Code: public class Main { public static void main(String[] args) { double myDouble = 9.5d; int myInt = (int)myDouble; System.out.println(myDouble); System.out.println(myInt); } }