Password in general is used to protect our information from third-party access. So, if we have the requirement to create a password python helps us to create password.
According to the requirement we can ask the compiler to print the password with lowercase, uppercase and special characters.
The harder the password generated the harder to crack it.
So python helps us to fulfill this requirement.
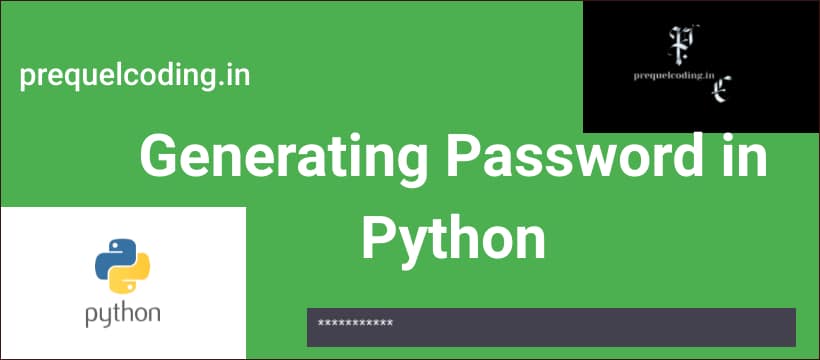
Now let us implement the code..
importing library: import random Why to import random? Random module contains the random numerical digits required for the code.. Now let us declare a variable to print the length of the password. password_length = int(input("Ener the length of password: ")) Now let us try to invoke lowercase, uppercase, special characters and numericals in our code in array. UPPERCASE = ['A', 'B', 'C', 'D', 'E', ] LOWERCASE = ['a', 'c', 'd', 'e', 'f'] DIGITS = ['1', '3', '5', '7', '9'] SPECIAL = ['@', '#', '$', '*'] Now we need to combine all the arrays in one form. COMBINED_LIST = DIGITS + UPPERCASE + LOWERCASE + SPECIAL password = " " .join(random.sample(COMBINED_LIST, password_len)) Why this line? We are going to join the function created our password to an empty string on left. Now finally print the password. print(password) Full Code: import random password_len = int(input("Enter length of password: ")) UPPERCASE = ['A', 'B', 'C', 'D', 'E', ] LOWERCASE = ['a', 'c', 'd', 'e', 'f'] DIGITS = ['1', '3', '5', '7', '9'] SPECIAL = ['@', '#', '$', '*'] COMBINED_LIST = DIGITS + UPPERCASE + LOWERCASE + SPECIAL password = " " .join(random.sample(COMBINED_LIST, password_len)) print(password)
//ouput:
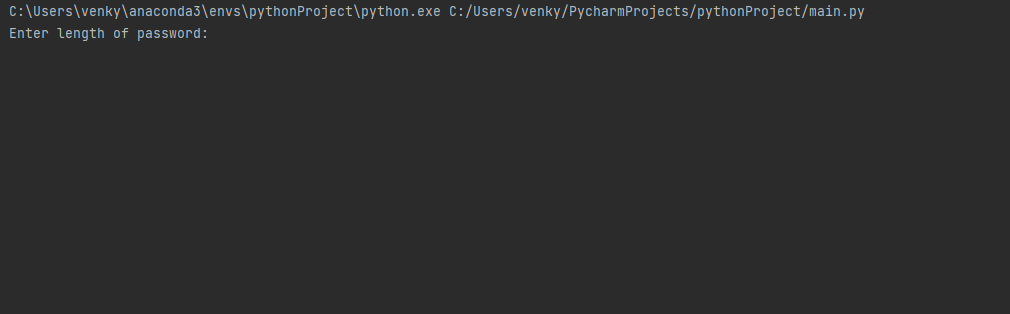
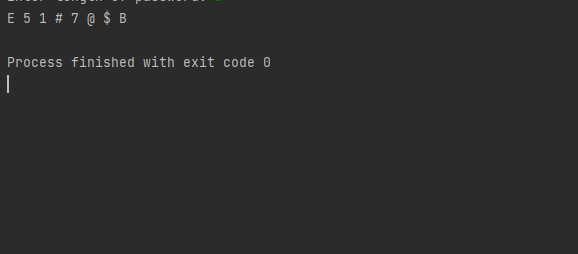