How to compare two strings in C?
Comparing Two Strings:
- Generally, to compare two numbers or any condition to be achieved in C programming we use (==) operator but by using it we cannot compare two strings so in this scenario because using that operator will compare the reference of the string but not the strings.
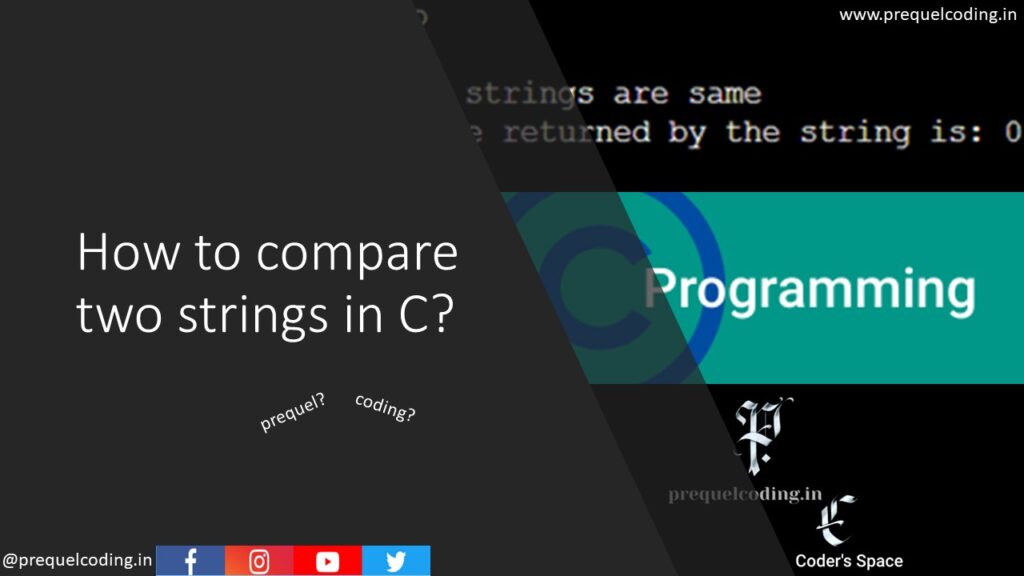
- What we do?
- In this case we use the strcmp() string function which will compare two strings.
- In this you will have three cases in which you can compare your strings:
- When they are equal your result will be zero.
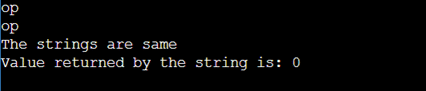
- When the first-string characters are matching with that of the second string then a positive value is returned.

- When the first-string characters and the second-string characters are completely different then it returns a negative value.

- Now let us see the coding part and understand it more clearly.
Code:
//Including libraries and definition of the main function. #include<stdio.h> #include<string.h> int main() { //Declaring variables: char str1[40]; char str2[100]; //Reading inputs from the user: printf("The first string is: "); scanf("%s",str1); printf("The second string is: "); scanf("%s",str2); int res; //Declaring our result to the comparison function: res = strcmp(str1,str2); // Looping conditions: if(res==0){ printf("The strings are same"); } else{ printf("They are not same"); } //Resulting value of our string: printf("\nValue of the resulted string is: %d",res); } //Whole code: #include<stdio.h> #include<string.h> int main() { char str1[40]; char str2[100]; printf("The first string is: "); scanf("%s",str1); printf("The second string is: "); scanf("%s",str2); int res; res = strcmp(str1,str2); if(res==0){ printf("The strings are same"); } else{ printf("They are not same"); } printf("\nValue of the resulted string is: %d",res); }