Copying a String:
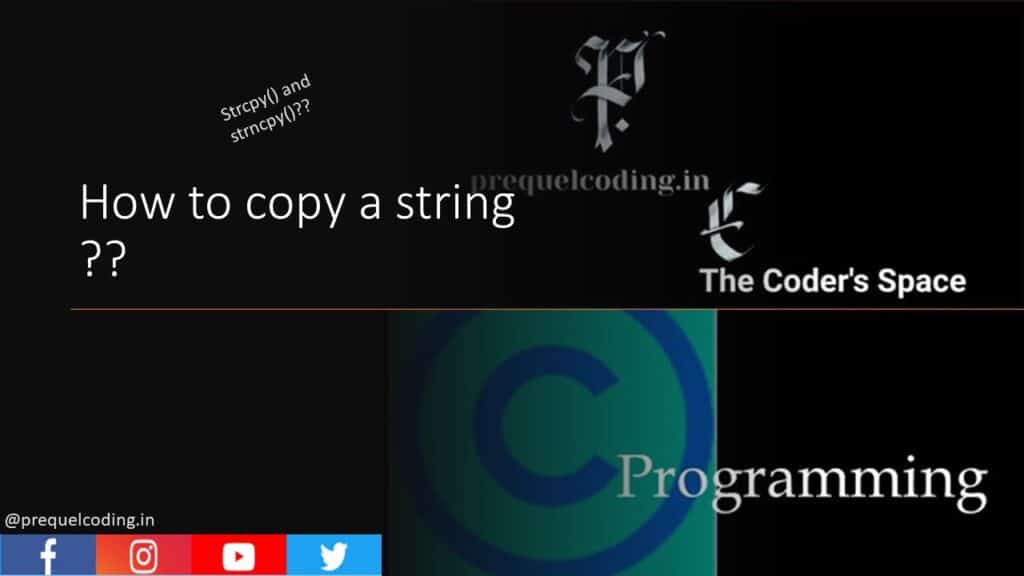
- Since you cannot assign arrays in C, you cannot assign strings either.
- char s[100]; //declare
- s = “hello”; // initialize – DOESN’T WORK (‘lvalue required’ error)
- you can also use an strncpy() function to copy a string to an existing string.
- The string equivalent of the assignment operator.
char src[50], dest[50];
strcpy(src, “This is source”);
strcpy(dest, “This is destination”);
- The strcpy() function doesn’t check to see whether the source string actually fits in the target string
- Safer way to copy strings is to use strncpy()
- Strncpy() takes the third argument.
- The maximum number of characters to copy.
#include <stdio.h> #include<string.h> int main() { char src[30] = "Prequelcoding is coder space"; char temp[50]; strcpy(temp, src); printf("The channel: %s\n", temp); return 0; }

If we want to print a upto a certain range then:
#include <stdio.h> #include<string.h> int main() { char src[30] = "Prequelcoding is coder space"; char temp[50]; //size of the array which is going to hold the source or src strncpy(temp, src, 10); temp[10] = '\0'; //we are going to do the null termination after 10 characters. printf("The channel: %s\n", temp); return 0; }
